This snippet monitors dynamic changes on the WooCommerce cart page and automatically hides non-free shipping options when free shipping is selected
before without script
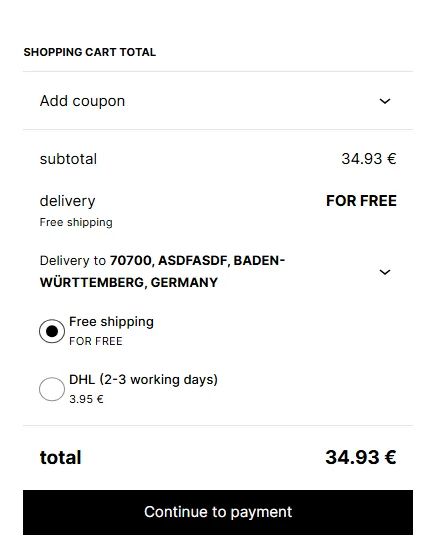
after adding the script auto hides display:none the other flat rate
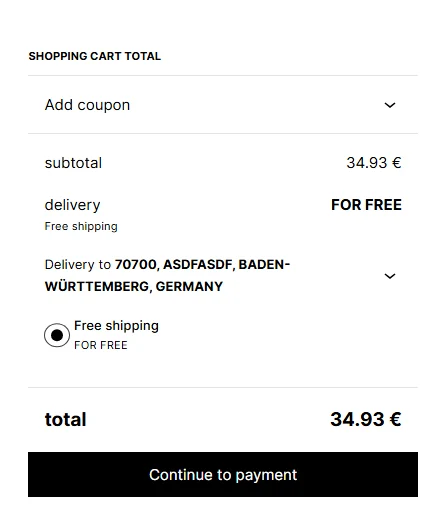
<script> // Function to check for free shipping and hide non-free options function checkFreeShipping() { // Find all shipping option containers on the cart page var shippingContainers = document.querySelectorAll('.wc-block-components-radio-control'); if (!shippingContainers.length) return; // Exit early if container(s) don't exist shippingContainers.forEach(function(container) { // Get all the shipping option labels within the container var labels = container.querySelectorAll('label'); var freeShippingSelected = false; // First, check if any free shipping option is selected. labels.forEach(function(label) { var input = label.querySelector('input[type="radio"]'); if (input && input.value.indexOf('free_shipping') !== -1) { // Check if the input is selected or if its parent label has the "checked" class if (input.checked || label.classList.contains('wc-block-components-radio-control__option-checked')) { freeShippingSelected = true; } } }); // If free shipping is selected, hide all non-free shipping options. if (freeShippingSelected) { labels.forEach(function(label) { var input = label.querySelector('input[type="radio"]'); if (input && input.value.indexOf('free_shipping') === -1) { label.style.display = 'none'; } }); } else { // Otherwise, ensure all shipping options are visible. labels.forEach(function(label) { label.style.display = ''; }); } }); } // Set up a mutation observer on the shipping container function observeShippingContainer(container) { var observer = new MutationObserver(function(mutationsList) { checkFreeShipping(); }); observer.observe(container, { childList: true, subtree: true }); } // Wait for the shipping container to be added if not present initially function waitForShippingContainer() { var container = document.querySelector('.wc-block-components-radio-control'); if (container) { observeShippingContainer(container); checkFreeShipping(); return; } // If container is not found, observe the document body for additions var bodyObserver = new MutationObserver(function(mutationsList, obs) { var container = document.querySelector('.wc-block-components-radio-control'); if (container) { observeShippingContainer(container); checkFreeShipping(); obs.disconnect(); } }); bodyObserver.observe(document.body, { childList: true, subtree: true }); } // Use event delegation to listen for changes on shipping radio inputs. // This ensures that even if the DOM changes dynamically the event listener remains active. document.body.addEventListener('change', function(event) { if (event.target && event.target.matches('.wc-block-components-radio-control input[type="radio"]')) { // Slight delay to allow asynchronous UI updates setTimeout(checkFreeShipping, 100); } }); // Initialize when the DOM is ready document.addEventListener('DOMContentLoaded', function() { checkFreeShipping(); waitForShippingContainer(); }); </script>